Getting started with Network Automation using Python and Netmiko pt II
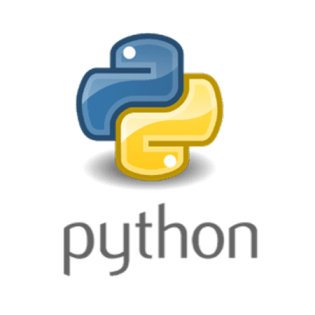
Let’s get started where we left off from part one.. With an upstream and downstream reading from the router, rather crudely extracted from a line of text output and placed into a pair of variables.
So, previously we were able to print the bandwidth values prepended by some text. This time we will wrap some text around these variables to create meaningful IOS commands ready for delivery to our device.
Let’s start where we generated screen output from last time :
print ("bandwidth",upstream)
print ("bandwidth receive",downstream)
We can use this text and the variables to create a new string to build each command we’ll be sending to the router :
upstream_cli = "bandwidth " + upstream
downstream_cli = "bandwidth receive " + downstream
Now we need to make some preparations to send these to the router by placing them into a list.
cli_commands = ['interface dialer 0', upstream_cli, downstream_cli]
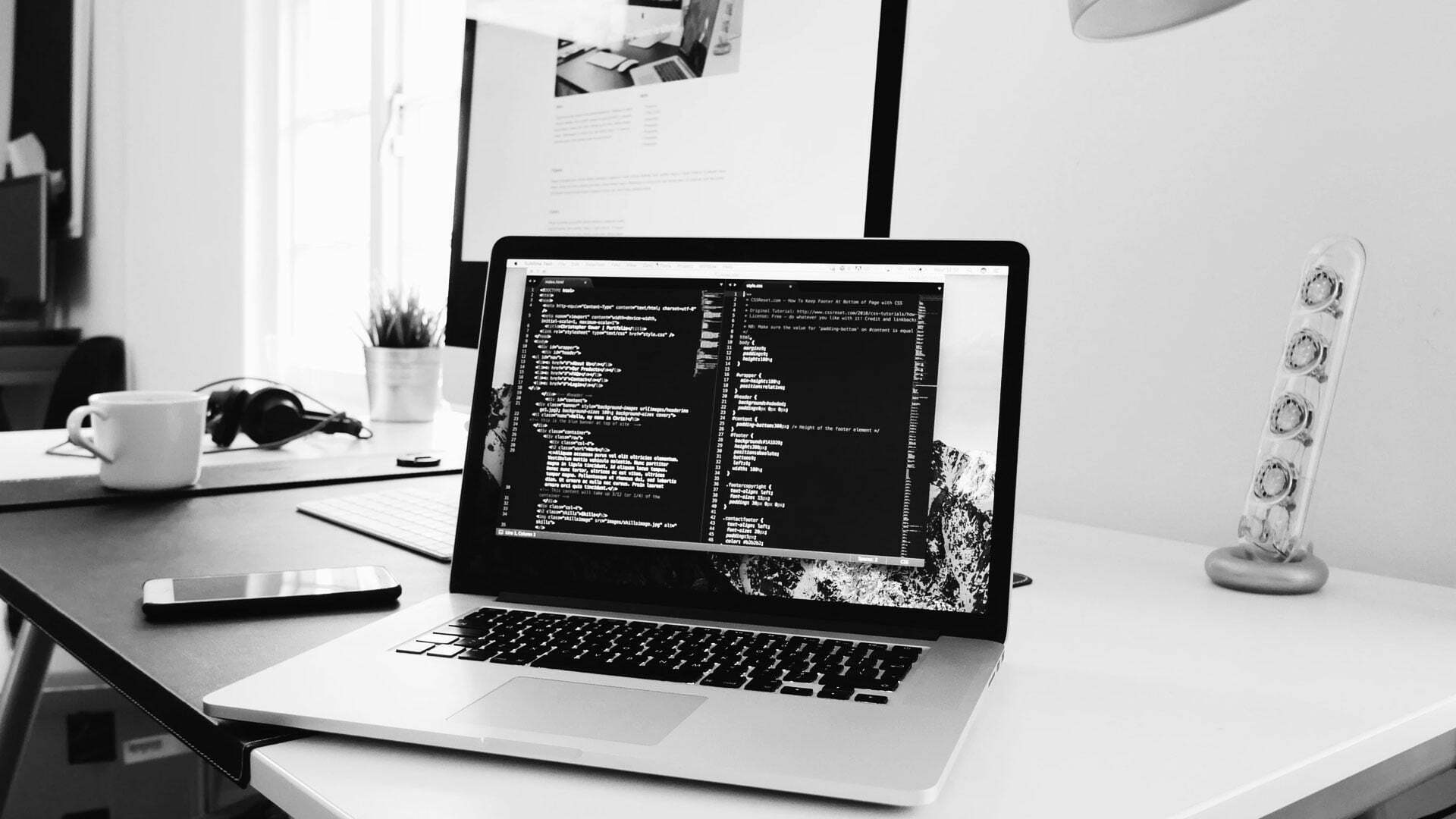
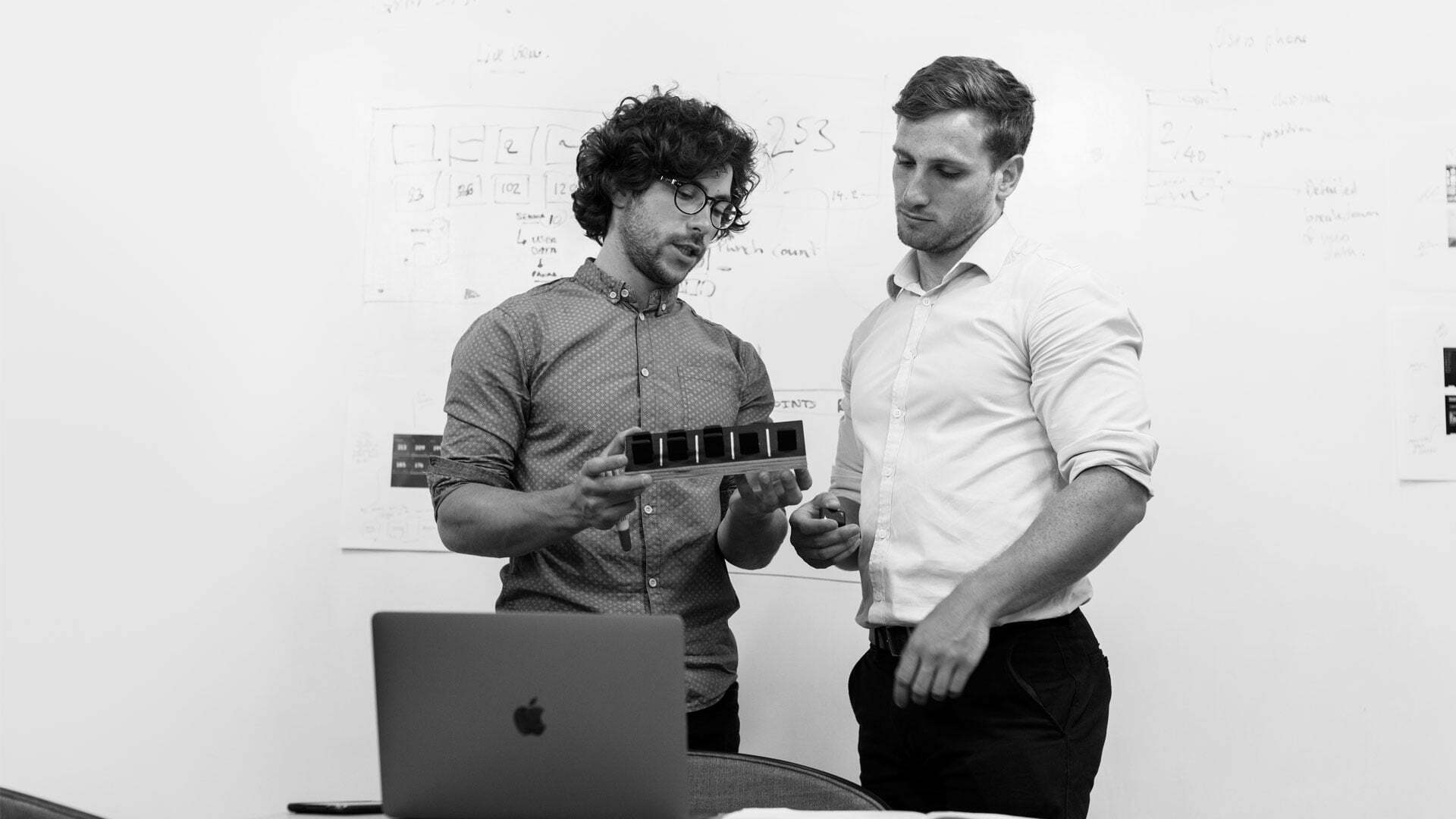
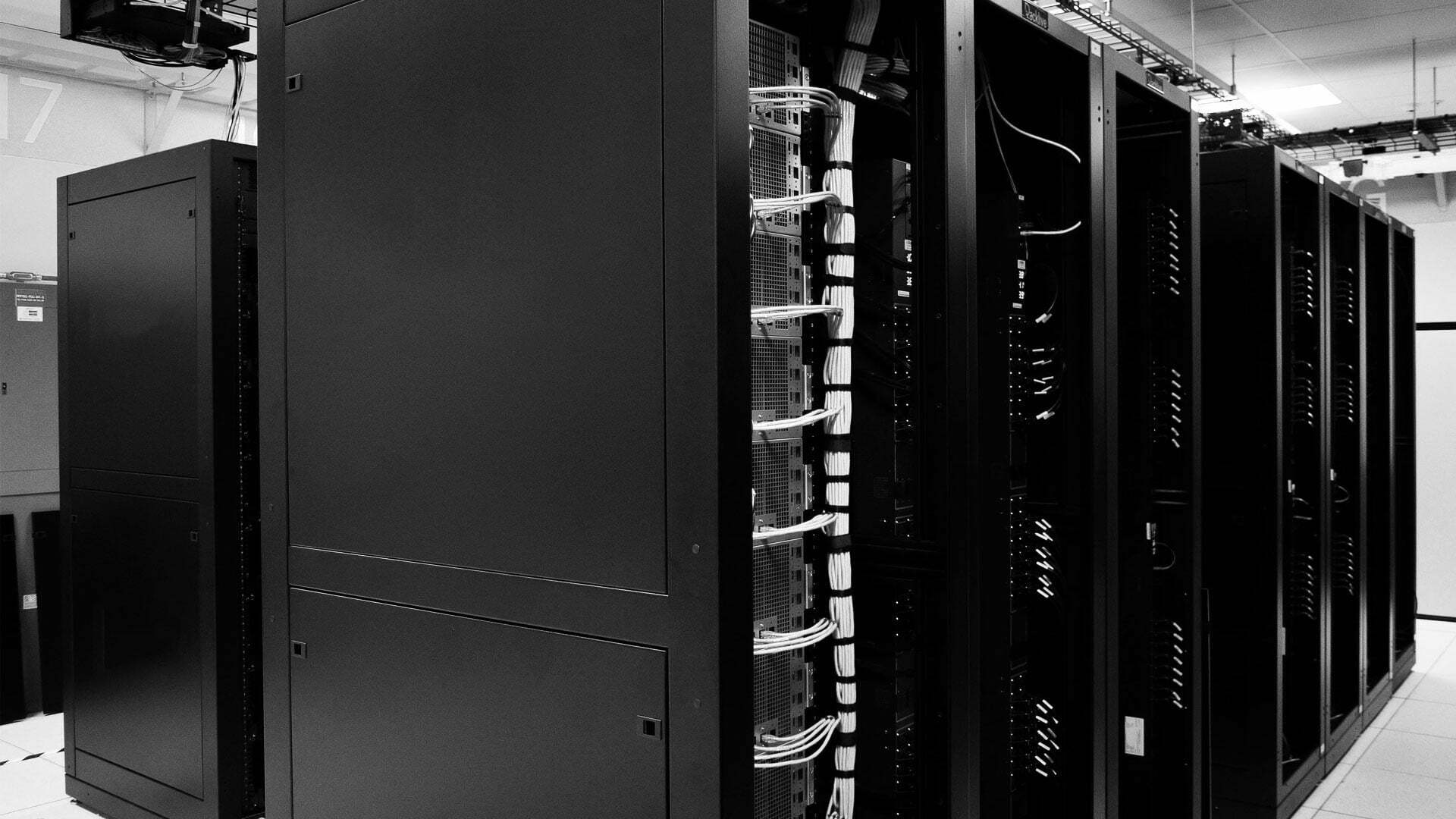
We have created a list, which Netmiko will iterate over and deliver to the router in order. We get this done by passing the list to Netmiko’s send_config_set method. Remember that we already setup the ssh connection via net_connect to the router earlier in part 1, which should still be active.
Recall that Netmiko already knows we are configuring a Cisco device, so it helpfully adds the required ‘conf t’ as it delivers the commands for us.
net_connect.enable() # enter enable mode
net_connect.send_config_set(cli_commands) # send the commands from our list
net_connect.disconnect() # close the connection gracefully
And there we have it, our first automated configuration change is complete. If we log onto the router, a ‘show run int dial 0’ verifies that our commands have been successfully delivered.
interface Dialer0
bandwidth 760
bandwidth receive 4347
snip-
For reference, here is the finished Python code :
from netmiko import ConnectHandler
router = {
'device_type': 'cisco_ios',
'ip': 'IP_ADDRESS',
'username': 'USERNAME',
'password': 'PASSWORD',
'port' : 22, # optional, defaults to 22
'secret': 'ENABLE PASSWORD', # optional, defaults to ''
'verbose': True, # optional, defaults to True
}
net_connect = ConnectHandler(**router)
output = net_connect.send_command('show controllers vdsl 0')
output = output.split('n')
print ("parsing output..n")
for line in output:
if '(kbps)' in line:
speed = line
print (line,'n')
speed = speed.split(' ')
downstream = str(speed[19])
upstream = str(speed[37])
upstream_cli = "bandwidth " + upstream
downstream_cli = "bandwidth receive " + downstream
cli_commands = ['interface dialer 0', upstream_cli, downstream_cli]
for line in cli_commands:
print (line)
net_connect.enable()
net_connect.send_config_set(cli_commands)
net_connect.disconnect()
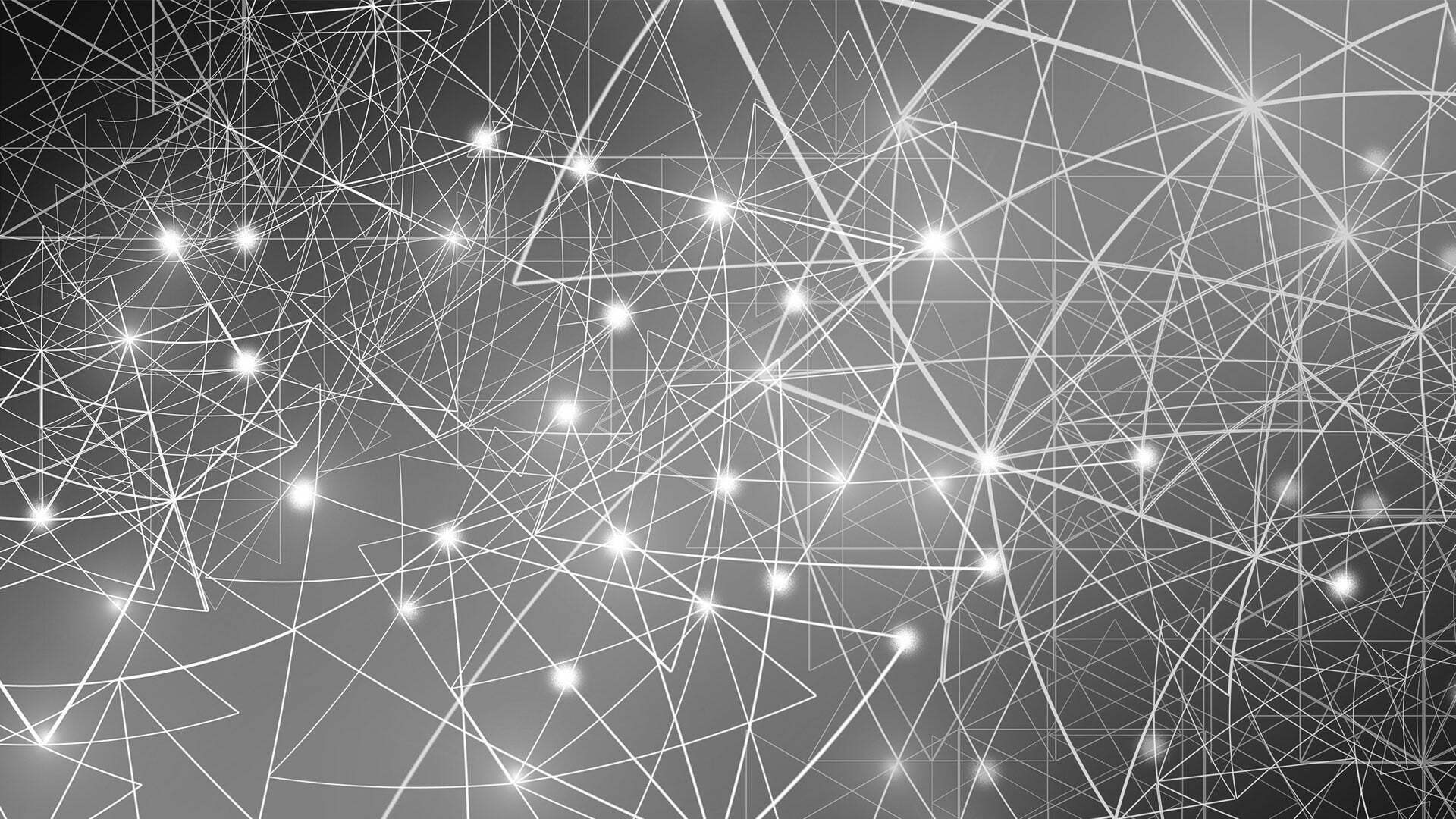