Getting started with Network Automation using Python and Netmiko
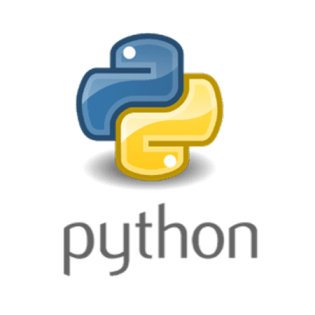
A couple of years back, when I was just getting started on my network automation journey..
I struggled for a long time with telnet libraries like telnetlib and even resorted to dropping into a CLI SSH process and scraping output via pexpect. To say it was cumbersome and error-prone is an understatement. I eventually gave up and went back to CLI bashing and using Kiwi / Solarwinds Cattools for delivering bulk changes.
That was until I discovered Netmiko, which provides a clean and reliable way to connect to network devices and return output. Netmiko is a Python library designed handle all the logistics and nuances of connecting to network devices’ CLI interfaces programatically, particularly where no native interface such as REST or NETCONF is available. It’s not all about scraping output either, this library does a great job of delivering configuration changes too.
Netmiko extends Paramiko (a Python library for managing SSH connections) by adding support for network devices and is written by Kirk Byers. As with all good open source projects, source code can be checked out from GitHub, or installed easily using Python’s pip installer.
I opted for the pip install on OSX, which is incredibly simple :
- $pip install netmiko
- Collecting netmiko
- Downloading netmiko-0.2.5-py2.py3-none-any.whl
- Installing collected packages: netmiko
- Successfully installed netmiko-0.2.5
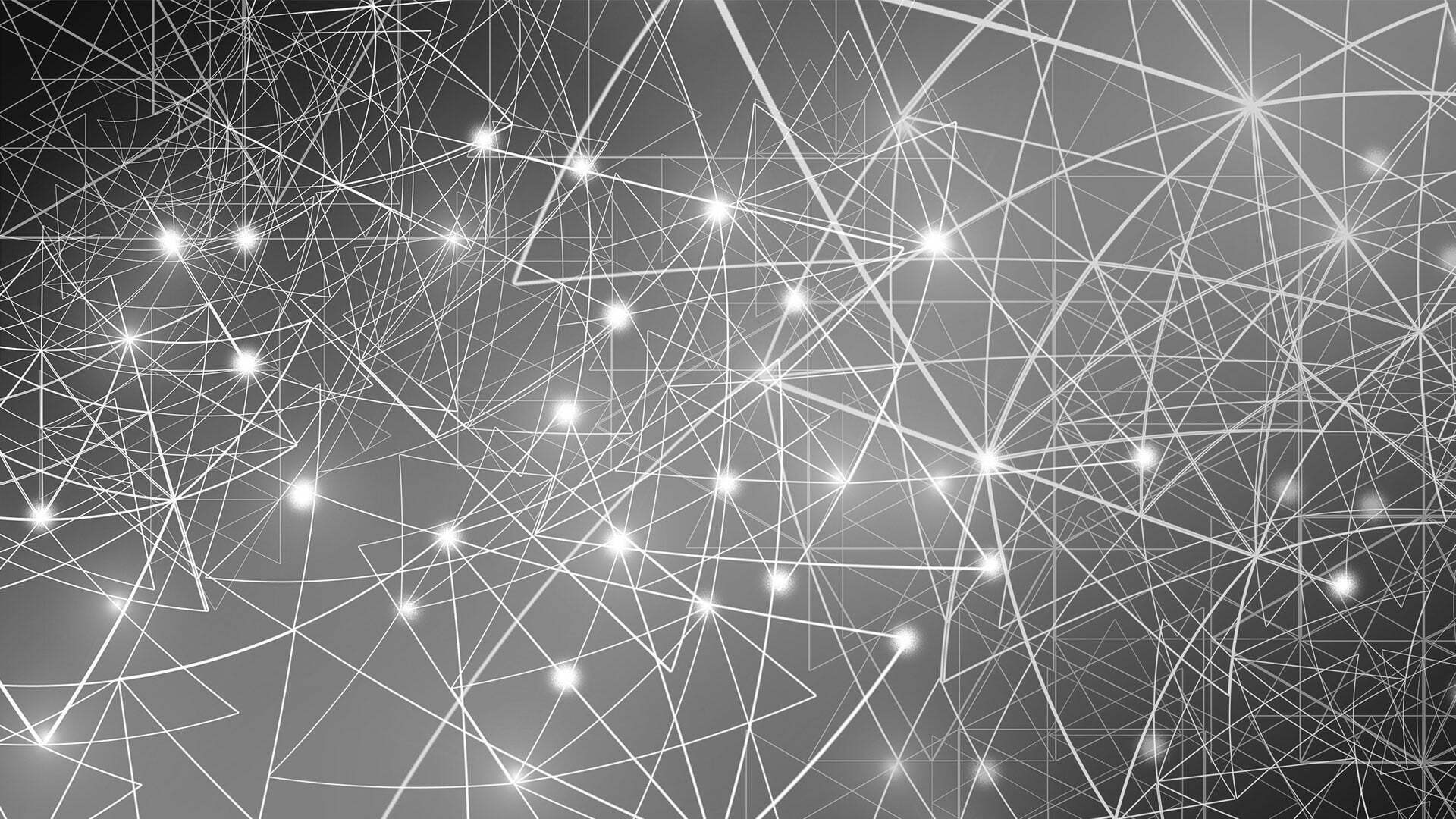
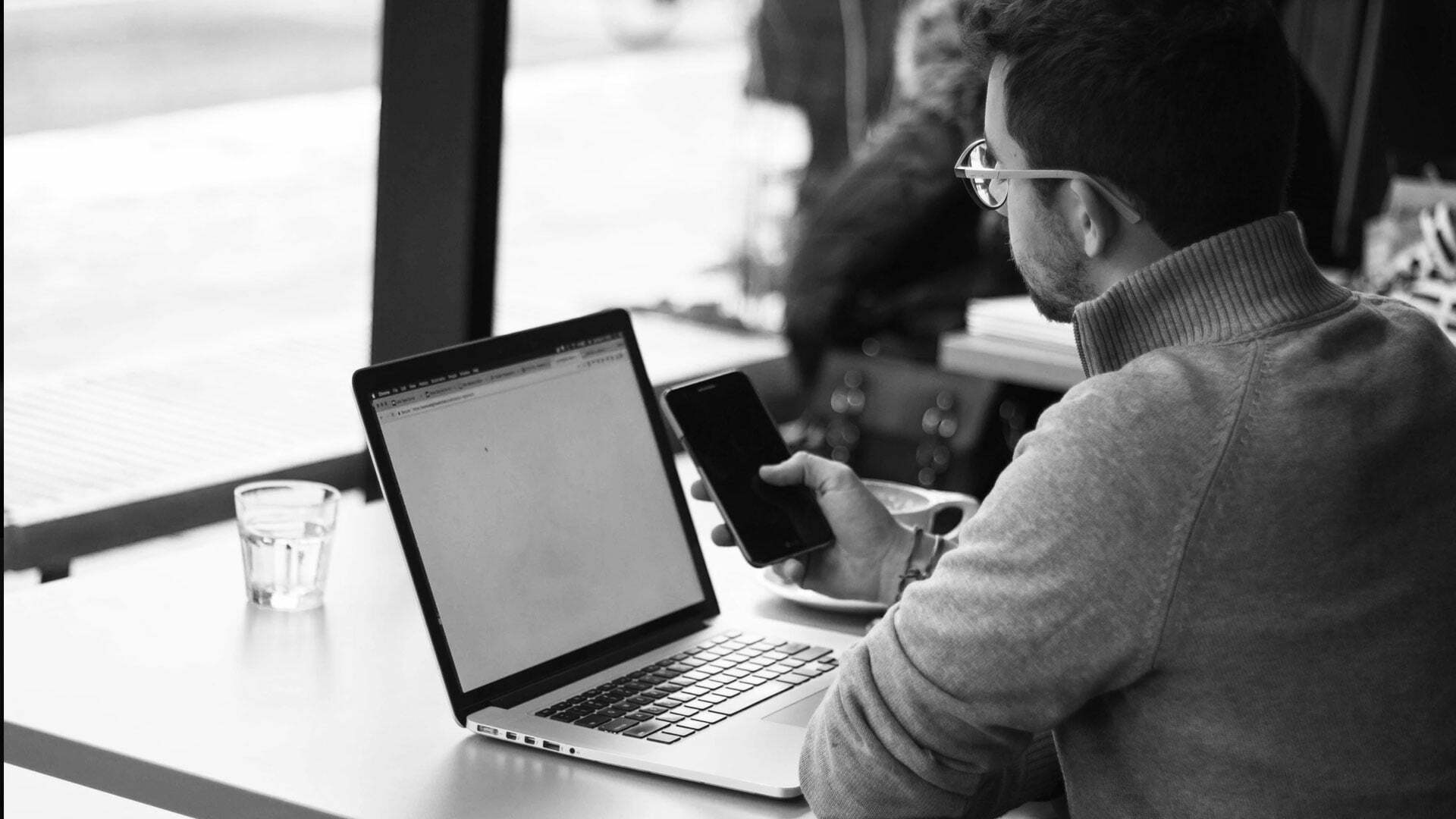
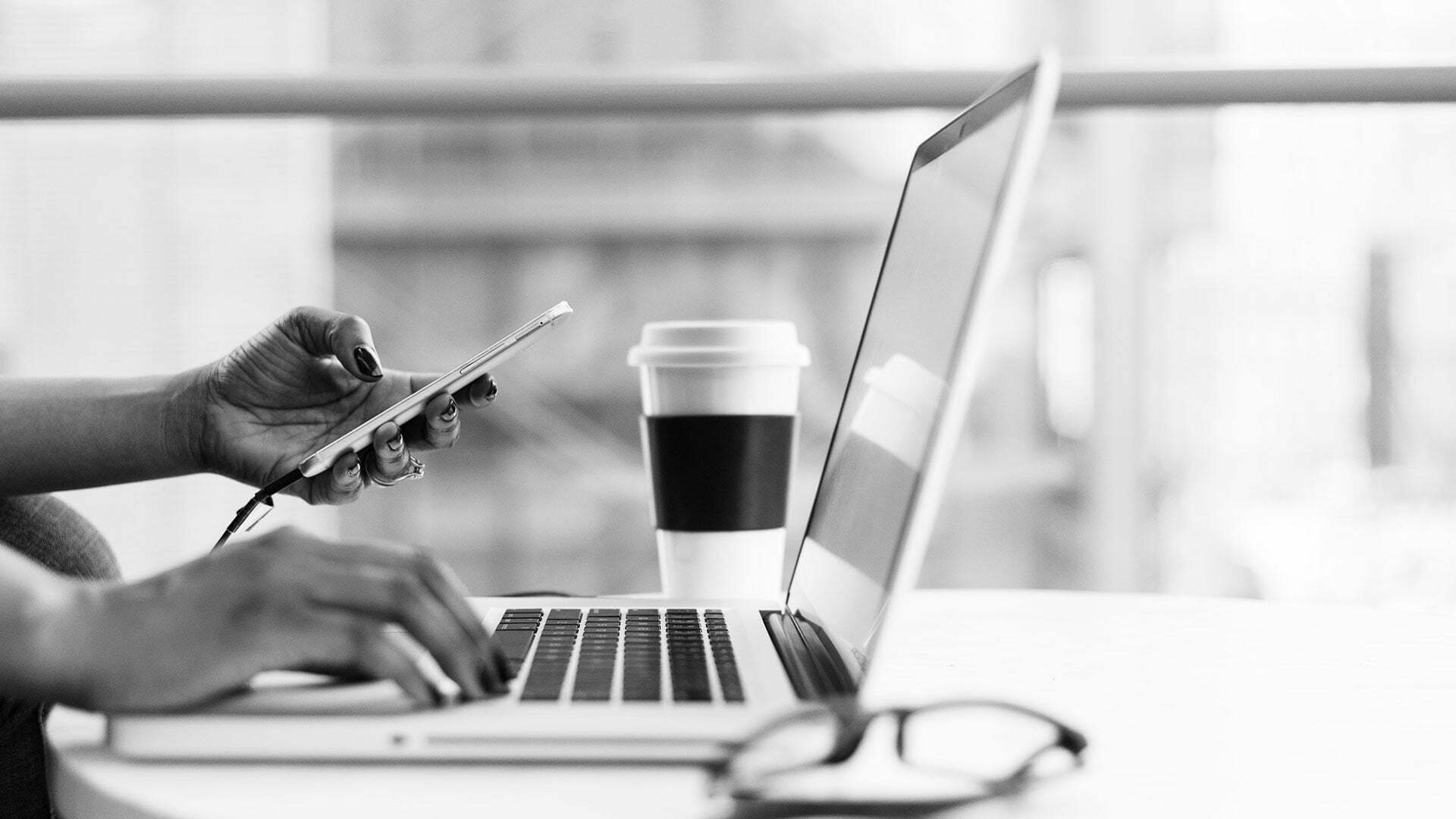
There are several ways to install pip on my OS of choice MacOS, some examples can be found on StackExchange(https://stackoverflow.com/questions/17271319/installing-pip-on-mac-os-x).
To get started with a basic example, load up the Python interpreter and import the Netmiko libary :
$python
Python 2.7.10 (v2.7.10:15c95b7d81dc, May 23 2015, 09:33:12)
[GCC 4.2.1 (Apple Inc. build 5666) (dot 3)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> from netmiko import ConnectHandler
From here we can easily connect to a Cisco IOS device (or Juniper Junos, Dell Force10, Fortinet etc) and collect some basic DSL line statistics :
router = {
'device_type': 'cisco_ios',
'ip': '1.2.3.4',
'username': 'USERNAME',
'password': 'PASSWORD',
'port' : 22, # optional, defaults to 22
'secret': 'ENABLE', # optional, defaults to ''
'verbose': True, # optional, defaults to True }
net_connect = ConnectHandler(**router)
output = net_connect.send_command('show controllers vdsl 0')
output = output.split('n')
#output is returned as a single string, so let's split this into lines in a list for iteration later
for line in output:
if '(kbps)' in line:
speed = line
print (line,'n')
#for debugging purposes, let us know when we see the (kbps) line
If we separate whitespace on the line we’ve found, we can locate the upstream and downstream speeds :
speed = speed.split(' ')
downstream = str(speed[19]) #list entry 19 = downstream speed
upstream = str(speed[37]) #list entry 37 = upstream speed
print ("bandwidth",upstream)
print ("bandwidth receive",downstream)
Output from this script :
$ python3 dsl.py
SSH connection established to 1.2.3.4:22
Interactive SSH session established
parsing output..
Speed (kbps): 0 4347 0 760
bandwidth 760
bandwidth receive 4347
Now, it would be trivial take these variables and use them to generate some configuration, dropping them back onto the relevant dialer interface using the net_connect.send_config_set method, helping monitoring tools like Solarwinds Orion report the correct interface speeds for DSL interfaces, rather than the defaults we usually see. I’ll save that for my next post though..
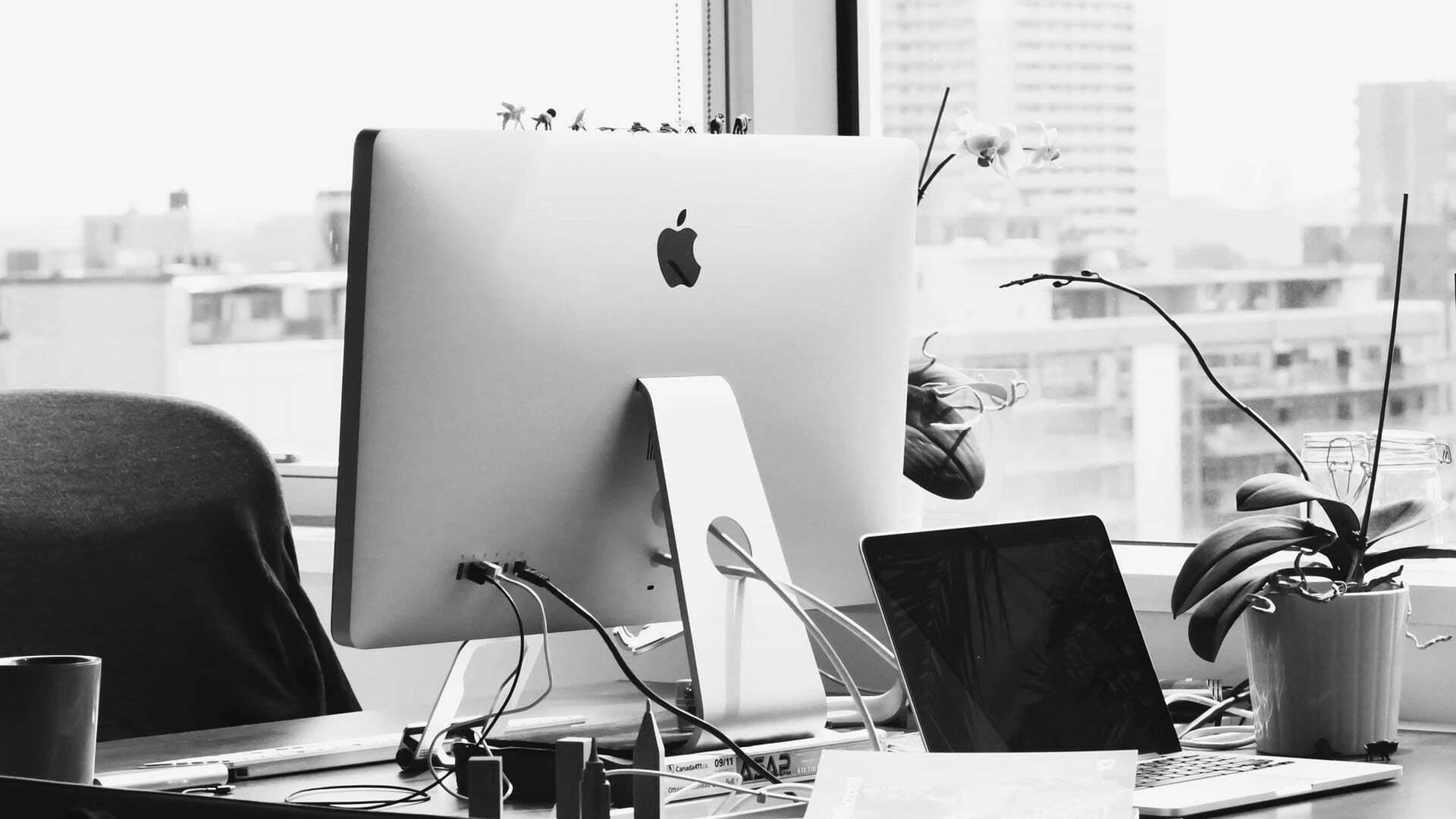